其中数据库操作使用了Microsoft.ApplicationBlocks,请参考我的《Microsoft.ApplicationBlocks使用心得》 类结构图:
相关文件: 接口:IManager.cs IPlanManager.cs 实现:Manager.cs PlanManager.cs 使用:Test.cs 其他相关文件不予说明 Test.cs:测试客户端,可以进行单个功能类内多个方法的事务控制,如果要进行多个功能类的事务控制就需要修改基类Manager中数据库连接的获得方式,目前是简单的获取连接串后直接创建连接,实用时候应该从一个数据库连接工厂类中获得,这样多个Manager类可以共享数据库连接,进行事务控制,并可以有效地管理数据库连接池。
using System;
using System.Collections;
using ReceptionPlan.Data.Components;
using ReceptionPlan.Data.IDAL;
using ReceptionPlan.Data.DAL;
namespace ReceptionPlan.Data.Services
{ 
/**//// <summary>
/// ReceptionPlan 的摘要说明。
/// </summary>
public class ReceptionPlanService
{
public ReceptionPlanService()
{
}
/**//// <summary>
/// 添加计划
/// </summary>
/// <param name="plan">计划对象</param>
/// <returns>计划ID</returns>
public static int AddPlan(Plan plan)
{
IPlanManager pm = new PlanManager();
pm.BeginTransaction();
try
{
int id = pm.AddPlan(plan);
plan.Id = id;
plan.Name = "XXXX";
pm.ModifyPlan(plan)
pm.Commit();
return id;
}
catch(Exception ex)
{
pm.Rollback();
throw ex;
}
}
}
IManager.cs
using System;
namespace ReceptionPlan.Data.IDAL
{ 
/**//// <summary>
/// IManager 的摘要说明。
/// </summary>
public interface IManager
{
void BeginTransaction();
void Rollback();
void Commit();
}
}
IPlanManager.cs
using System;
using System.Collections;
using ReceptionPlan.Data.Components;
namespace ReceptionPlan.Data.IDAL
{ 
/**//// <summary>
/// IPlanManager 的摘要说明。
/// </summary>
public interface IPlanManager : IManager
{
int AddPlan(Plan plan);
int ModifyPlan(Plan plan);
}
}
Manager.cs
using System;
using System.Data;
using System.Data.SqlClient;
using System.Collections;
using ReceptionPlan.Data.IDAL;
using ReceptionPlan.Data.Utility;

namespace ReceptionPlan.Data.DAL
{ 
public abstract class Manager : IManager
{
protected SqlTransaction trans;
protected SqlConnection conn;
protected ArrayList connList;

public Manager()
{
connList = new ArrayList();
}

~Manager()
{
if (trans != null)
trans.Rollback();

/**//*
foreach(SqlConnection temp in connList)
{
if(temp !=null && temp.State != ConnectionState.Closed )
temp.Close();
}*/
}
//public SqlConnection Connection
public string Connection
{ 
get
{
return Configs.CONN_STR;
/**//*
SqlConnection temp = new SqlConnection(Configs.CONN_STR);
connList.Add(temp);
return temp;
*/
}
}

public void BeginTransaction()
{
conn = new SqlConnection(Configs.CONN_STR);
conn.Open();
trans = conn.BeginTransaction();
}

public void Rollback()
{ 
if (trans != null)
{
trans.Rollback();
trans = null;
conn.Close();
}
}

public void Commit()
{ 
if (trans != null)
{
trans.Commit();
trans = null;
conn.Close();
}
}
}
}
PlanManager.cs
using System;
using System.Collections;
using Microsoft.ApplicationBlocks.Data;
using System.Data;
using System.Data.SqlClient;
using ReceptionPlan.Data.Components;
using ReceptionPlan.Data.IDAL;
using ReceptionPlan.Data.Utility;
namespace ReceptionPlan.Data.DAL
{ 
/**//// <summary>
/// PlanManager 的摘要说明。
/// </summary>
public class PlanManager : Manager, IPlanManager
{
public PlanManager()
{
}
public int AddPlan(Plan plan)
{
int id = -1;
string sql = string.Empty;
try
{
sql = string.Format("insert into [RP_Plan] XXXX);
object AID = SqlHelper.ExecuteScalar(trans, CommandType.Text, sql );
id = Convert.ToInt32(AID);
}
catch(Exception ex)
{
throw new Exception(ex.ToString() + "\n sql=" + sql);
}
return id;
}
public int ModifyPlan(Plan plan)
{
if (plan.Id < 0)
return -1;
string sql = string.Empty;
int ret = -1;
try
{
sql = string.Format("update RP_Plan XXXX);
ret = SqlHelper.ExecuteNonQuery(trans, CommandType.Text, sql );
}
catch(Exception ex)
{
throw new Exception(ex.ToString() + "\n sql=" + sql);
}
return ret;
}
}
}
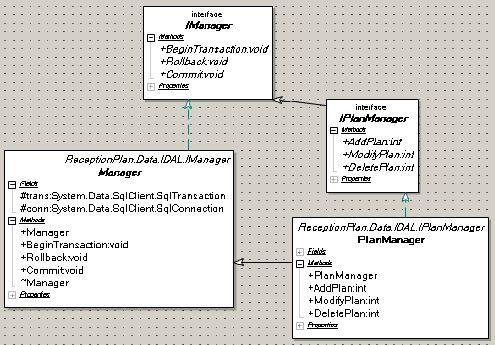

































































































































































































































































